How to build a MCP server for your ai chatbot.
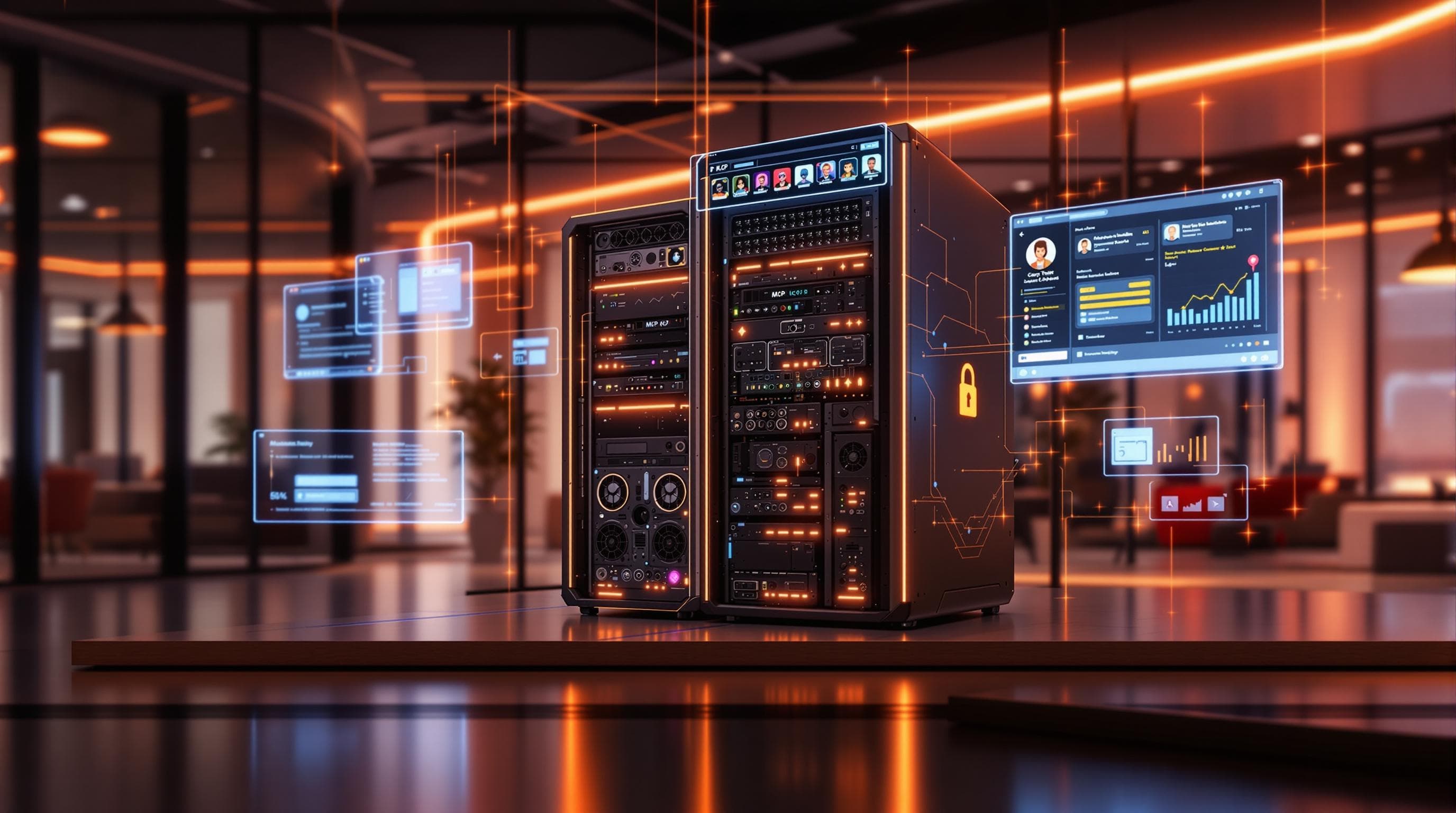
How to build a MCP server for your ai chatbot.
Want to build a smarter AI chatbot? Start with an MCP server.
An MCP (Message Control Protocol) server is the backbone of a high-performance chatbot. It manages messages, tracks conversation context, and integrates with AI models like OpenAssistantGPT. Here's why you need it:
- Faster responses with caching and smart request handling
- Better security through input validation and rate limiting
- Scalability to handle growing user demands
- Detailed logging for debugging and performance tracking
Key Steps to Build Your MCP Server:
- Set up your environment: Install Node.js, Git, and required packages.
- Configure API keys: Securely integrate OpenAI and OpenAssistantGPT APIs.
- Build server components: Include a request handler, context manager, and API integration layer.
- Add security: Implement rate limiting, input validation, and error handling.
- Test and deploy: Use health checks and diagnostic tools to ensure reliability.
Quick Overview of Benefits:
Feature | Benefit | Example |
---|---|---|
Smart Caching | Faster response times | Handles high traffic |
Input Sanitization | Protects against attacks | Prevents misuse |
Scalable Design | Grows with your chatbot | Adapts to more users |
Building an MCP server ensures your AI chatbot is fast, secure, and scalable. Ready to get started? Let’s dive in!
MCP Server Basics
MCP Server Definition
An MCP server is the backbone of a high-performance AI chatbot. It manages the flow of messages and keeps track of conversation context. By processing incoming messages, maintaining context, and connecting with OpenAssistantGPT, it ensures accurate and efficient responses. The server also validates incoming data and manages responses effectively.
Why Use MCP Servers
MCP servers bring several key benefits that make them a must-have for professional chatbot setups:
Performance Benefits:
- Faster response times thanks to smart caching
- Streamlined message handling, even during high traffic
- Efficient memory usage for extended conversations
Security Advantages:
- Protects against injection attacks through input sanitization
- Prevents misuse with rate limiting
- Safeguards API keys with secure management
Operational Improvements:
- Logs conversations in detail for analysis
- Monitors performance in real time
- Offers advanced tools for debugging
Main MCP Server Parts
An MCP server is built around three main components:
1. Request Handler
- Validates and organizes incoming messages
- Formats outgoing responses
- Manages traffic flow for both incoming and outgoing data
2. Context Manager
- Keeps track of conversation history
- Allocates memory efficiently
- Handles context windows to maintain coherence
3. API Integration Layer
- Manages API authentication
- Handles formatting for requests and responses
- Includes error-handling and retry mechanisms
These components work together to ensure smooth message processing. Once these elements are planned out, you can start setting up your development environment.
Preparing Your Workspace
Required Software
To get started with your MCP server, make sure you have the following tools installed:
- Node.js (v20.0.0 or higher)
- Git
- A code editor like VS Code
- A terminal application
- Your OpenAI API key
OpenAssistantGPT Setup
Set up OpenAssistantGPT to ensure it integrates smoothly with MCP. Begin by creating an account and selecting a plan that fits your needs. Here's what to configure:
Component | What to Do |
---|---|
API Access | Generate API keys from the OpenAssistantGPT dashboard. |
SDK Setup | Install the NextJS-based SDK using npm. |
Authentication | Set up SAML/SSO for Pro or Enterprise plans. |
Environment | Define all necessary environment variables. |
Once this setup is complete, you can move on to preparing your local development environment.
Development Environment Setup
Follow these steps to configure your local workspace:
1. Install Dependencies
Start by creating a project directory and initializing it:
mkdir mcp-server
cd mcp-server
npm init -y
2. Set Up Environment Variables
In the root of your project, create a .env
file and add the following:
OPENAI_API_KEY=your_api_key_here
OPENASSISTANT_API_KEY=your_key_here
PORT=3000
NODE_ENV=development
3. Install Required Packages
Run the following command to install necessary packages:
npm install @openassistantgpt/sdk express dotenv
To get the most out of your development workflow, consider adding these extensions to your code editor:
- ESLint for maintaining code quality
- Prettier for consistent code formatting
- GitLens to enhance version control
- Thunder Client for testing APIs
With everything set up, you're ready to dive into building your MCP server!
Building Your MCP Server
Setting Up the Server Base
Start by creating the main application file (server.js
) to set up your server:
const express = require('express');
const dotenv = require('dotenv');
const { OpenAssistantGPT } = require('@openassistantgpt/sdk');
dotenv.config();
const app = express();
app.use(express.json());
const port = process.env.PORT || 3000;
const assistant = new OpenAssistantGPT({
apiKey: process.env.OPENASSISTANT_API_KEY
});
app.listen(port, () => {
console.log(`MCP Server running on port ${port}`);
});
Once the base server is ready, you can integrate the OpenAI API to handle dynamic responses.
OpenAI API Integration
Add a /chat
route to process API requests:
app.post('/chat', async (req, res) => {
try {
const response = await assistant.createChat({
model: 'gpt-4',
messages: req.body.messages,
temperature: 0.7
});
res.json(response);
} catch (error) {
res.status(500).json({ error: error.message });
}
});
Here’s a quick reference table for choosing the right model:
Model Option | Best Use Case | Message Limit |
---|---|---|
GPT-4 | Complex reasoning | 8,192 tokens |
GPT-3.5 | General queries | 4,096 tokens |
GPT-4o | Vision tasks | 128,000 tokens |
Server Testing Guide
To ensure everything is working, add these testing routes:
app.get('/health', (req, res) => {
res.status(200).json({
status: 'healthy',
timestamp: new Date().toISOString()
});
});
app.post('/test', async (req, res) => {
try {
const testMessage = { role: 'user', content: 'Test message' };
const response = await assistant.processMessage(testMessage);
res.json(response);
} catch (error) {
res.status(500).json({ error: error.message });
}
});
Once testing confirms the server works as expected, you can move on to securing and deploying it.
Launch Preparation
Before going live, implement these security measures:
const rateLimit = require('express-rate-limit');
const limiter = rateLimit({
windowMs: 15 * 60 * 1000,
max: 100
});
app.use(limiter);
const helmet = require('helmet');
app.use(helmet());
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).json({ error: 'Internal server error' });
});
Make sure all required environment variables are set:
const requiredEnvVars = [
'OPENASSISTANT_API_KEY',
'NODE_ENV'
];
requiredEnvVars.forEach(varName => {
if (!process.env[varName]) {
throw new Error(`Missing required environment variable: ${varName}`);
}
});
Lastly, add a diagnostic endpoint to monitor server performance:
app.get('/metrics', (req, res) => {
const metrics = {
uptime: process.uptime(),
memory: process.memoryUsage(),
cpu: process.cpuUsage()
};
res.json(metrics);
});
With these steps, your server will be secure, functional, and ready for deployment.
sbb-itb-7a6b5a0
Linking Server to Chatbot
OpenAssistantGPT Connection
To connect your MCP server with OpenAssistantGPT, use the following setup:
const { OpenAssistantGPT } = require('@openassistantgpt/sdk');
const chatbotConfig = {
apiKey: process.env.OPENASSISTANT_API_KEY,
chatbotId: process.env.CHATBOT_ID,
maxTokens: 2048,
temperature: 0.7
};
const chatbot = new OpenAssistantGPT(chatbotConfig);
app.post('/connect', async (req, res) => {
try {
const connection = await chatbot.initialize();
res.json({
status: 'connected',
sessionId: connection.sessionId
});
} catch (error) {
res.status(500).json({
error: 'Connection failed',
details: error.message
});
}
});
Message Processing
Once the connection is active, you can manage sessions and track context by implementing a message processing endpoint:
const messageQueue = new Map();
app.post('/process-message', async (req, res) => {
const { sessionId, message } = req.body;
try {
messageQueue.set(sessionId, {
timestamp: Date.now(),
status: 'processing'
});
const response = await chatbot.processMessage({
sessionId,
message,
context: {
previousMessages: req.body.history || [],
userPreferences: req.body.preferences || {}
}
});
messageQueue.delete(sessionId);
res.json(response);
} catch (error) {
messageQueue.delete(sessionId);
res.status(500).json({
error: 'Processing failed',
details: error.message
});
}
});
Server Speed Improvements
To enhance your server's performance, consider caching responses and processing messages in batches:
const cache = require('node-cache');
const messageCache = new cache({ stdTTL: 600 });
app.use(async (req, res, next) => {
const cacheKey = `${req.path}-${JSON.stringify(req.body)}`;
const cachedResponse = messageCache.get(cacheKey);
if (cachedResponse) {
return res.json(cachedResponse);
}
res.sendResponse = res.json;
res.json = (body) => {
messageCache.set(cacheKey, body);
res.sendResponse(body);
};
next();
});
const batchProcessor = {
queue: [],
processing: false,
batchSize: 5,
async process() {
if (this.processing || this.queue.length === 0) return;
this.processing = true;
const batch = this.queue.splice(0, this.batchSize);
try {
const responses = await Promise.all(
batch.map(item => chatbot.processMessage(item.message))
);
batch.forEach((item, index) => {
item.resolve(responses[index]);
});
} catch (error) {
batch.forEach(item => item.reject(error));
}
this.processing = false;
this.process();
}
};
Recommended Server Settings
To maintain reliability and efficiency, configure your server with these settings:
Setting | Value | Purpose |
---|---|---|
Max Concurrent Connections | 1,000 | Prevents server overload |
Response Timeout | 30 seconds | Ensures timely responses |
Cache TTL | 600 seconds | Reduces API calls |
Batch Size | 5 messages | Optimizes processing |
Memory Limit | 1.5 GB | Maintains stability |
Build a Real-world MCP Server with One TypeScript File | Full ...
Server Management Tips
To keep your MCP server running efficiently, effective server management is essential. Below, you'll find practical methods to maintain a secure and scalable environment.
Cache and Request Limits
Optimize your server's performance by implementing caching and request limits. Here's a sample setup using Redis:
const rateLimit = require('express-rate-limit');
const RedisStore = require('rate-limit-redis');
const limiter = rateLimit({
store: new RedisStore({
host: 'localhost',
port: 6379,
expiry: 60 * 15 // 15 minutes
}),
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per windowMs
message: 'Too many requests, please try again later'
});
app.use('/api/', limiter);
Adjust caching rules based on the type of request to ensure optimal performance. Here's a quick guide:
Request Type | Cache Duration | Invalidation Trigger |
---|---|---|
User Queries | 10 minutes | New Training Data |
System Status | 5 minutes | Config Changes |
Analytics | 30 minutes | Manual Refresh |
Static Assets | 24 hours | Version Update |
Error Management
A structured error-handling system is crucial for logging issues and providing appropriate responses. Here's an example:
const errorHandler = async (error, context) => {
const errorLog = {
timestamp: new Date().toISOString(),
errorType: error.name,
message: error.message,
stack: error.stack,
context: context
};
// Log to monitoring system
await logger.error(errorLog);
// Determine response based on error type
switch (error.name) {
case 'ValidationError':
return {
status: 400,
message: 'Invalid input parameters'
};
case 'AuthenticationError':
return {
status: 401,
message: 'Authentication required'
};
default:
return {
status: 500,
message: 'Internal server error'
};
}
};
Keep an eye on these critical metrics for smooth operation:
- Response time anomalies (over 500ms)
- Failed authentication attempts
- Memory usage spikes (above 85%)
- API rate limit breaches
Integrating these practices will help stabilize performance while preparing for new features.
Adding New Features
Feature flags are a great way to control the rollout of new capabilities. Here's an example:
const featureFlags = {
enableNewFeature: async (feature) => {
return {
name: feature,
enabled: process.env.ENABLE_FEATURES.includes(feature),
config: await loadFeatureConfig(feature)
};
},
loadFeatureConfig: async (feature) => {
const config = await ConfigService.get(feature);
return {
maxRequests: config.maxRequests || 1000,
timeout: config.timeout || 5000,
retryAttempts: config.retryAttempts || 3
};
}
};
Key components for rolling out new features:
Component | Purpose | Implementation Guide |
---|---|---|
Feature Flag | Control rollout | Use environment variables |
Monitoring | Track usage | Use Prometheus metrics |
Rate Limiting | Prevent abuse | Redis-based limits |
Error Handling | Manage failures | Define custom error types |
Documentation | Provide guidelines | Use OpenAPI/Swagger |
Conclusion
Setting up your MCP server effectively is key to building a reliable and scalable conversational interface for your AI chatbot. A well-configured MCP server ensures smooth message processing, efficient performance, and proper error handling.
Research shows that implementing AI chatbots can lead to a 35% drop in support tickets and a 60% faster resolution time, potentially saving businesses up to $25,000 annually. These outcomes depend heavily on a properly configured MCP server with the right features in place.
Here’s a quick breakdown of the critical components for a successful MCP server setup:
Component | Impact | Best Practice |
---|---|---|
Server Architecture | Performance | Monitor resource usage regularly |
Error Management | Reliability | Use proven error-handling techniques |
Feature Integration | Scalability | Implement features with feature flags |
Message Processing | Efficiency | Focus on optimizing response times |
The platform offers flexible plans to match your needs, starting with a Free Plan (500 monthly messages) and scaling up to the Enterprise Plan with unlimited capabilities. This structure ensures your chatbot can grow alongside your requirements.
To maintain top-notch performance, keep an eye on server metrics and tweak configurations as needed based on usage patterns. This proactive approach ensures consistent service quality and maximizes the benefits of AI-driven functionality.
"Build your AI chatbot with no code." - OpenAssistantGPT